Part 1 | Set Cookie's
Overview:
In this new module, we are going to add in guest checkout capabilities. This means that a user visiting the site will be able to add items to cart and checkout without ever needing to create an account.
Our site visitors will also have the ability to leave our website and still have their items in cart on their next visit.
Using Cookies
We will be using the visitors browser to create cookies. I won't go into details about cookies because there is tons of information online. But, essentially, it's just a way to store information in a users browser associated with a certain webpage.
Try opening up your console right now and finding where the cookies are stored. You should be able to find them by opening up your console (Right Click --> Inspect element), then opening the "application" tab.
If you look in my screenshot, you should see a cookie called "cart" with curly braces as the value. This is what we will be creating and how we will store cart data for non-logged in users.
Step 1 | Create Cart
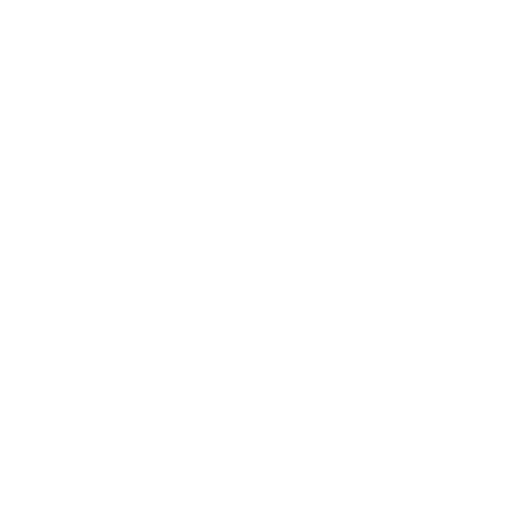
When a visitor comes to our website, we want to create a cookie for our cart. Regardless of whether they are logged in or not.
In our "main.html" file, within the script tag, I am going to add in a function that will search for and parse a specific cookie that is stored in our browser.
Once we have the function, we are going to search for a cookie called "cart", and if we cannot find one we are going to create + set it.
Get Cookie
First. let's start by adding the getCookie() function into the script tag just underneath our csrf token, you can find it in the source code or code block.
Don't worry about what the function does for now. I did some research and just copied a cookie parser function myself so honestly, I can't say I know too much about it other than the fact that it works.
Once we set the function, let's set the variable "cart" to the return value of "cart" from our cookie parser.
Create Cookie
If our browser does not contain a cookie called "cart", which is expected on the first load, let's create one.
First check the status of cart, then set cart to an empty Javasctript object and use document.cookie to set one.
A cookie is a key-value part so we set the name with "cart=" and then stringify the Javascript object (cart), then set the domain so we have this same cookie on every page.
If we don't add ";domain=;path/" then we will create a new cookie on each page and our cart will NOT be consistent, so be sure to add this.
View Source CodeStep 2 | Adding/Removing Items
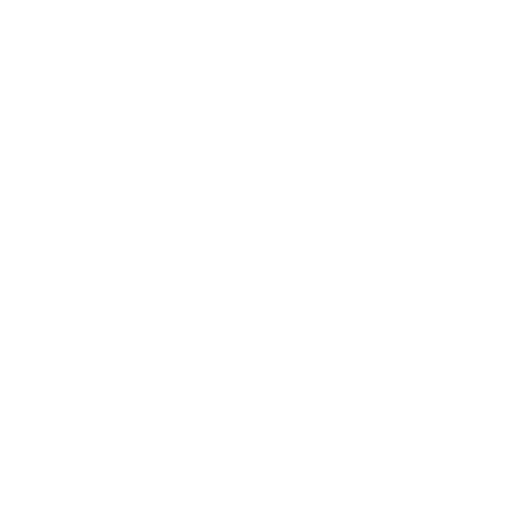
Several steps back in module #3 we created an add to cart feature in "cart.js" which sent a post request and added items to our database.
On "click" there was an "if" statement that checked the users status and at this point we essentially ignored clicks made by users that were not logged in. We want to create a function that will be triggered on the first condition.
Add to cookie item function
Let's create a function below our "updateUserOrder" funciton in cart.js called "addCookieItem()".
This function, just like updateUserOrder(), will take in the "productId" and "action" as parameters and will be triggered on the first condition within our "if" statement.
If you want to ensure everything is working, be sure to log out and test by clicking the "add" button and checking the console.
Update Cookie
Inside our addCookieItem() function, let's add some logic that determines what happens to our cart.
First, we want to check the "action" type to know if we need to add or remove an item.
Add or Increase item Quantity
If the action is "add", we want to check if this item is already in the cart. If not, then we create it. If we already have that Item in our cart, we simply want to add to the quantity.
Our cookie object will contain nested objects with the id of the product being the id and the quantity.
Remove or Decrease item Quantity
If the action is "remove", we want to either decrease the quantity, or, if the quantity is equal to or below zero, remove it from our cart altogether.
Change cookie value in browser
Finally we want to actually update the browsers cookie because so far, we have just been working with a Javascript object.
To do this, we simply use document.cookie like we did in main.html and set our cookie while stringifying it.
Go ahead and test this out by adding some items and checking the cookies in your browser. If you're not seeing results, you may have to do a hard refresh with ctr+shift+r.
View Source Code