Part 4 | cookieCart() Function
Overview:
At this point we have taken our browsers cart cookie and made a full cart (Order) with cart items (OrderItem). The problem with this is we have about 30 lines of code that we will have to be using in multiple views. We need to use this data in multiple pages and that can make our code messy.
Let's make our code reusable
To stick with the concept of D.R.Y. (Don’t Repeat Yourself), let's be good programmers and build one function that creates this cart for us and just call it in each view to keep things clean.
And to keep things extra clean we will build this function in its own folder and simply import it into our views.
Step 1 | utils.py
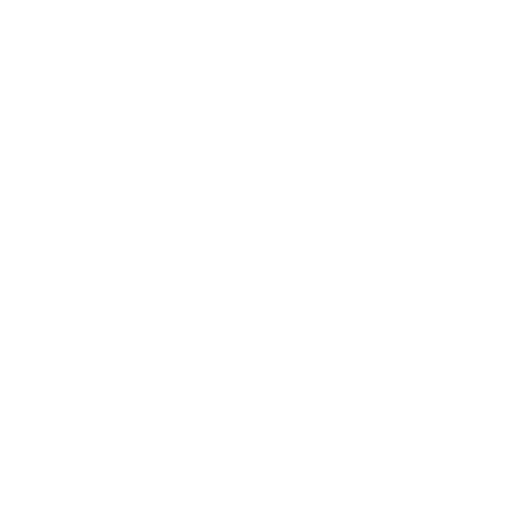
Inside our app we are going to create a new file to store some helper functions that we are going to create.
Create new file | utils.py
Let's create a new file called "utils.py" inside our app. Let's import json and our models into our new file.
import json
from .models import *
Create Function | cookieCart()
Create a function called cookieCart(). The purpose of this function is to handle all the logic we created for our guest users order.
This function will take in "request" as a parameter.
Import cookieCart() into views.py
from .utils import cookieCart
View Source CodeStep 2 | Copy Cart Logic
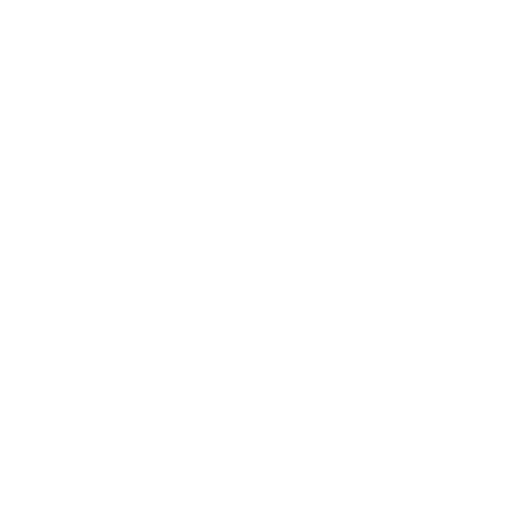
Let's copy all of the logic that generates our cart from the browsers cookies and paste it to the new cookieCart function inside our utils.py.
views.py
Once we've pasted this logic into our cookieCart function; update the "return" value to the following:
utils.py
View Source CodeStep 3 | User cookieCart in views
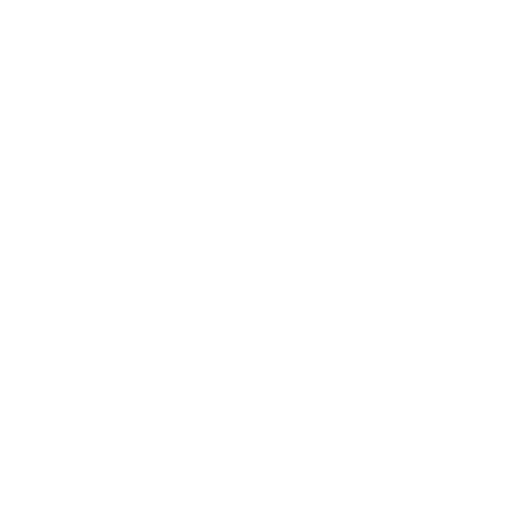
To make use of our new cookieCart funciton; let's clear out all of the logic in the "else" condition in "if request.user.is_authenticated:" and call our cookieCart function.
We will set the return value to the variable "cookieData" and now we can access the values returned by the function,
{'cartItems':cartItems ,'order':order, 'items':items}
We can then access these values from "cookieData" and get what we need for each view.
Now we have the same exact data as before by accessing the function with MUCH cleaner views. Adding this funciton and using its data as I showed will make our cart available from all pages now.
View Source Code