Part 6 | Checkout
Overview:
To close out the final part in this module we need to give the user the ability to checkout with their guest shopping cart. We need to do a few things in this section.
1. Clear cart in browsers cookies when users check out and redirect them from the checkout page.
2. Send cart data to the backend and create all necessary information for the customer and order.
Step 1 | Clear Cart
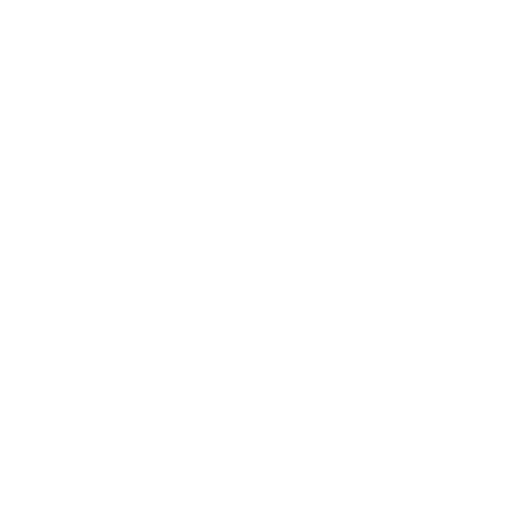
In the "checkout.html" pages Javascript; let's clear cart when our payment button/form is successfully submitted.
To do this we will set cart to an empty dictionary and update our cookie. We'll want to put this in the fetch calls "promise" so we only clear it when the data was properly submitted.
Also make sure to add it BEFORE we send the user back to our main page or the cart won't clear.
View Source CodeStep 2 | Guest Checkout Logic
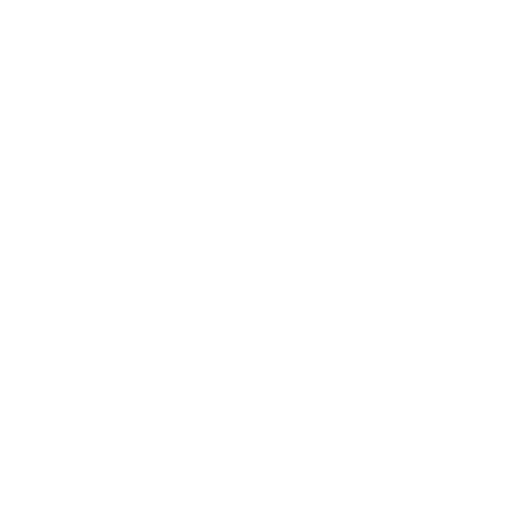
It's time to process our guests order when they submit the payment button. Right now we are sending data to our "processOrder" view but we are only handling logged in users.
Get cookie cart
Let's start by building our cart data using the cookie cart function. We will need to query our items and build a real order now.
Create customer & Order
We will use get_or_create for our customer. Even though the customer is checking out without an account we still want to add orders associated with emails and allow the customer to see all previous orders if they ever decide to create an account.
Create an order and set the value to the customer variable we just set. "Complete" should be set to "false" since this is an open cart until payment is confirmed and processed.
Create Items
Let's loop through our cart items replica list and create real OrderItems by querying the product and setting the nessesary attributes.
Move actions/Fix Indentation
There are a few lines of code that currently sit inside our "else" statement that need to be moved below our condition.
We need to move everything from "total" to "order.save()" below and outside of our else statement. Because we have created an "order" for both logged in and guest users we need to make this available for both conditions.
Pay close attention to the indentation of where I put the code block.
View Source CodeStep 3 | Create guest checkout function
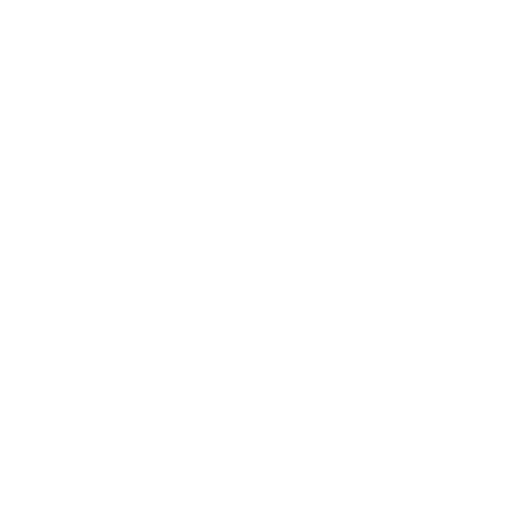
Like we've done so a couple of times aready, let's clean up this view by creating a function that takes care of creating an order for a "guest" and "returns" the order.
We'll call this function "guestOrder" and place it in "utils.py". We'll be copying the guest checking logic in here.
Create function (utils.py)
The function should take in "data" and "request" as parameters.
Copy/paste
Let's take all the logic inside our "else" condition in the "processOrder" view and paste it in to the new guestOrder function. the function should return our order.
View Source CodeStep 4 | Clean up processOrder View
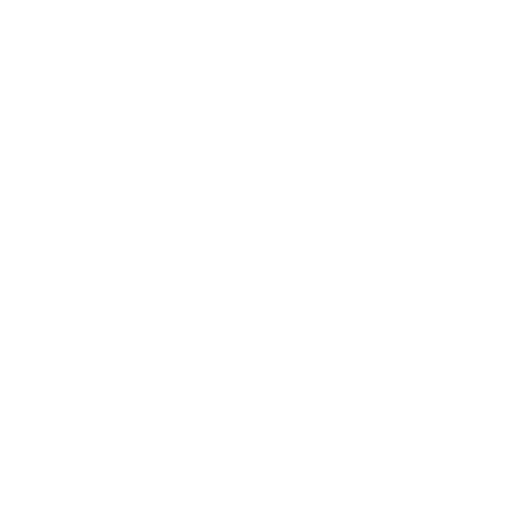
The last thing we need to take care of to actually use this function is to import and replace our else statement logic with our function.
Import function (views.py)
from .utils import cookieCart, cartData, guestOrder
Clean up view
We return and asign the values of "customer" and "order" in once call. Once we set this, test the website by logging in and out and processing a few orders.
View Source Code